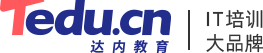
课程咨询: 400-996-5531 / 投诉建议: 400-111-8989
认真做教育 专心促就业
在Python中,计算一个数的立方根可以使用多种方法。以下是一些常见的方法,以及相应的代码示例。
### 方法一:使用``运算符
你可以使用``运算符和分数来表示立方根。立方根实际上是数的`1/3`次方。
```python
def cube_root(n):
return n (1/3)
# 使用示例
num = 27
print(f"The cube root of {num} is {cube_root(num)}")
```
### 方法二:使用`math`模块
Python的`math`模块提供了一个名为`pow`的函数,该函数可以接受三个参数:基数、指数和模(可选)。但是,对于立方根,更常见的做法是使用`math.pow`的前两个参数,或者更简单地使用``运算符。不过,`math`模块确实有一个名为`isqrt`的函数用于计算平方根,但没有直接用于计算立方根的函数。尽管如此,你可以通过`math.pow`来计算立方根。
```python
import math
def cube_root_math(n):
return math.pow(n, 1/3)
# 使用示例
num = 27
print(f"The cube root of {num} is {cube_root_math(num)}")
```
### 方法三:使用`cmath`模块(对于复数)
如果你的数可能是复数(即包含虚数部分),那么你可以使用`cmath`模块。这个模块和`math`模块类似,但它支持复数运算。
```python
import cmath
def cube_root_complex(n):
return cmath.pow(n, 1/3)
# 使用示例(对于实数)
num = 27
print(f"The cube root of {num} is {cube_root_complex(num)}")
# 使用示例(对于复数)
num_complex = 2 + 3j
print(f"The cube root of {num_